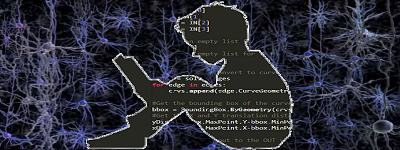
Jupyter Notebookなどで、コードを実装して実際に確かめてみましょう。
Jetson Nanoでjupyter-notebookを使う場合
mglearnは入ってないのでインストールしておきます
$sudo pip3 install mglearn
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 |
import numpy as np import matplotlib.pyplot as plt from sklearn.datasets import make_moons moons = make_moons(n_samples=200,noise=0.2,random_state=0) x = moons[0] y = moons[1] from matplotlib.colors import ListedColormap def plot_decision_boundary(model,x,y,margin=0.3): _x1 = np.linspace(x[:,0].min()-margin,x[:,0].max()+margin,100) _x2 = np.linspace(x[:,1].min()-margin,x[:,1].max()+margin,100) x1,x2 = np.meshgrid(_x1,_x2) x_new = np.c_[x1.ravel(),x2.ravel()] y_pred = model.predict(x_new).reshape(x1.shape) custom_cmap = ListedColormap(['mediumblue','orangered']) plt.contourf(x1,x2,y_pred,alpha=0.3,cmap=custom_cmap) def plot_dataset(x,y): plt.plot(x[:,0][y==0],x[:,1][y==0],'bo',ms=15) plt.plot(x[:,0][y==1],x[:,1][y==1],'r^',ms=15) plt.xlabel("$x_0$",fontsize=30) plt.ylabel("$x_1$",fontsize=30,rotation=0) plt.figure(figsize=(12,8)) plot_dataset(x,y) plt.show() ----------------------------------------------------- from sklearn.linear_model import LogisticRegression from sklearn.tree import DecisionTreeClassifier from sklearn.model_selection import train_test_split x_train,x_test,y_train,y_test = train_test_split(x,y,random_state=0) log_reg = LogisticRegression().fit(x_train,y_train) tree_clf = DecisionTreeClassifier().fit(x_train,y_train) print(log_reg.score(x_test,y_test)) print(tree_clf.score(x_test,y_test)) ----------------------------------------------------- from sklearn.model_selection import KFold,cross_val_score kfold = KFold(n_splits=5,shuffle=True,random_state=0) log_reg_score = cross_val_score(log_reg,x,y,cv=kfold) tree_clf_score = cross_val_score(tree_clf,x,y,cv=kfold) print(log_reg_score) print(tree_clf_score) print(log_reg_score.mean()) print(tree_clf_score.mean()) ----------------------------------------------------- from sklearn.metrics import confusion_matrix y_pred_log_reg = log_reg.predict(x_test) y_pred_tree_clf = tree_clf.predict(x_test) cm_log_reg = confusion_matrix(y_test,y_pred_log_reg) cm_tree_clf = confusion_matrix(y_test,y_pred_tree_clf) print(cm_log_reg) print(cm_tree_clf) ----------------------------------------------------- from sklearn.metrics import precision_score,recall_score,f1_score print("precision log_reg:\n",precision_score(y_test,y_pred_log_reg)) print("\n") print("precision tree_clf:\n",precision_score(y_test,y_pred_tree_clf)) print("recall log_reg:\n",recall_score(y_test,y_pred_log_reg)) print("\n") print("recall tree_clf:\n",recall_score(y_test,y_pred_tree_clf)) print("f1 log_reg:\n",f1_score(y_test,y_pred_log_reg)) print("\n") print("f1 tree_clf:\n",f1_score(y_test,y_pred_tree_clf)) ----------------------------------------------------- from sklearn.metrics import precision_recall_curve precision,recall,threshold = precision_recall_curve(y_test,y_pred_tree_clf) plt.figure(figsize=(12,8)) plt.plot(precision,recall) plt.xlabel("precision") plt.ylabel("recall") plt.show() precision,recall,threshold = precision_recall_curve(y_test,y_pred_log_reg) plt.figure(figsize=(12,8)) plt.plot(precision,recall) plt.xlabel("precision") plt.ylabel("recall") plt.show() ----------------------------------------------------- from mglearn.datasets import make_wave x,y = make_wave(n_samples=100) plt.figure(figsize=(12,8)) plt.scatter(x,y) plt.xlabel("x") plt.ylabel("y") plt.show() from sklearn.linear_model import LinearRegression x_train,x_test,y_train,y_test = train_test_split(x,y,random_state=0) lin_reg = LinearRegression().fit(x_train,y_train) print(lin_reg.score(x_test,y_test)) from sklearn.metrics import mean_squared_error y_pred = lin_reg.predict(x_test) mse = mean_squared_error(y_test,y_pred) mse rmse = np.sqrt(mse) rmse |
Leave a Reply