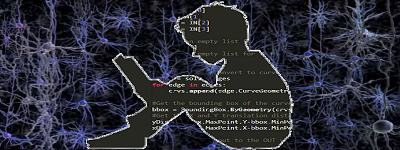
Jupyter Notebookなどで、コードを実装して実際に確かめてみましょう。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
import numpy as np import matplotlib.pyplot as plt from sklearn.datasets import make_blobs x,y = make_blobs(n_samples=200,n_features=2,centers=3,random_state=10) def plot_dataset(x,y): plt.plot(x[:,0][y==0],x[:,1][y==0],'bo',ms=15) plt.plot(x[:,0][y==1],x[:,1][y==1],'r^',ms=15) plt.plot(x[:,0][y==2],x[:,1][y==2],'gs',ms=15) plt.xlabel('$x_0$',fontsize=15) plt.ylabel('$x_1$',fontsize=15) plt.figure(figsize=(10,10)) plot_dataset(x,y) plt.show() #k-meansクラスタリング from sklearn.cluster import KMeans k_means = KMeans(n_clusters=3).fit(x) k_means.labels_ def plot_dataset(x,labels): plt.plot(x[:,0][labels==0],x[:,1][labels==0],'bo',ms=15) plt.plot(x[:,0][labels==1],x[:,1][labels==1],'r^',ms=15) plt.plot(x[:,0][labels==2],x[:,1][labels==2],'gs',ms=15) plt.xlabel('$x_0$',fontsize=15) plt.ylabel('$x_1$',fontsize=15) plt.figure(figsize=(10,10)) plot_dataset(x,k_means.labels_) plt.show() #別のデータでやってみる from sklearn.datasets import make_moons x,y = make_moons(n_samples=200,noise=0.1,random_state=0) plt.figure(figsize=(12,8)) plot_dataset(x,y) plt.show() k_means = KMeans(n_clusters=2).fit(x) k_means.labels_ plt.figure(figsize=(12,8)) plot_dataset(x,k_means.labels_) plt.show() ----------------------------------------------------- #階層的クラスタリング from sklearn.datasets import load_iris from scipy.cluster.hierarchy import dendrogram,linkage iris = load_iris() x = iris.data z = linkage(x,method='average',metric='euclidean') plt.figure(figsize=(18,12)) dendrogram(z) plt.show() |
Leave a Reply